Advanced Controls
Advanced controls allow you to configure more complex values for your stories.
These controls are not available in Flipbook
They are created with the provided constructors in the Utility Package
These are the currently available advanced controls:
Slider Control
Slider control allows you to add a number between a range, it gets displayed as a Slider with a number input, this control takes a min and max as the range

Slider(def, min, max, step? )
Arguments
- def number Default control value
- min number Minimum value range
- max number Maximum value range
- step numberOptional The increment/decrement step of the control, if not given the slider will be continuous Default: nil
RGBA Control
This control is similar to the Color3 primitive, but this one allows you to modify the alpha value of the color.
The alpha value gets converted to transparency when used in your story.
The control type will be: { Color:Color3, Transparency:number }

RGBA(def, transparency? )
Arguments
- def Color3 Default color value
- transparency numberOptional Default transparency value Default: 0
Choose Control
Choose control allows you to select between a set of options, it gets displayed as a dropdown with the options as the entries.
Possible values for this are: Tables, Datatypes, Enums, Functions, Primitives. You can mix types but this is not recommended.
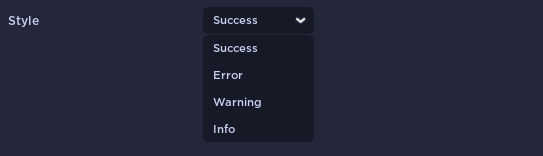
Choose(options, index? , widen? )
Arguments
- options Array Array of possible options
- index numberOptional Index of the default option Default: 1
- widen booleanOptional If true given, the control type will be widened (typescript only) Default: false
Enum List Control
Enum list control is similar to choose, but this one allows you to give a name to your options.
This is useful when the value itself doesn't provide enough information.
You can use the same types as Choose, and you can miix types, but this is, again, not recommended.
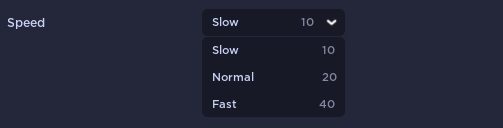
EnumList(list, key, widen? )
Arguments
- list { string: any } Record of possible options
- key keyof list Key of the default option, this is required Default: 1
- widen booleanOptional If true given, the control type will be widened (typescript only) Default: false
Widened Types
Choose and EnumList controls accept a third widen parameter.
This parameter doesn't change anything on runtime, but if true
is given, the control values will be generalized instead of the value literals
Example
Choose(["One", "Two", "Three"], 0, false); // type: "One" | "Two" | "Three"
Choose(["One", "Two", "Three"], 0, true); // type: string
Choose([1, 2, 3], 0, false); // type: 1 | 2 | 3
Choose([1, 2, 3], 0, true); // type: number
Example
Lets use all of them in a more real context:
local UILabs = require(...) -- Path of your Utility Package
local controls = {
-- [[ Choose ]] --
Theme = UILabs.Choose({"Dark", "Light"}),
Currency = UILabs.Choose({"Coins", "Gems"}),
-- [[ EnumList ]] --
WindowSize = UILabs.EnumList({
Mobile = 500,
Tablet = 1000,
Desktop = 1500,
}, "Mobile"),
TextColor = UILabs.EnumList({
Red = Color3.new(1, 0, 0),
Green = Color3.new(0, 1, 0),
Blue = Color3.new(0, 0, 1),
}, "Red"),
Volume = UILabs.Slider(50, 0, 100, 1), -- it only has integer values
FrameColor = UILabs.RGBA(Color3.new(1, 1, 1), 0) -- you will need to set BackgroundTransparency too
}
local story = {
controls = controls,
story = ...
}
return story